Do you want to make your own text-to-video or images-to-video generator tool?
Flickify is an excellent example that utilizes the concept of text-to-video and images-to-video tools. So, if you are interested in making a similar tool then this tutorial is for you.
Note: If you’re not familiar with Flickify then I’ll explain it to you. Basically, it is an online tool that converts articles/blog posts into videos. Flickify is part of Ezoic (a Google Adsense alternative for publishers to Increase Earnings, Improve Performance, & Grow Website Traffic Faster). You need a free Ezoic account to try Flickify.
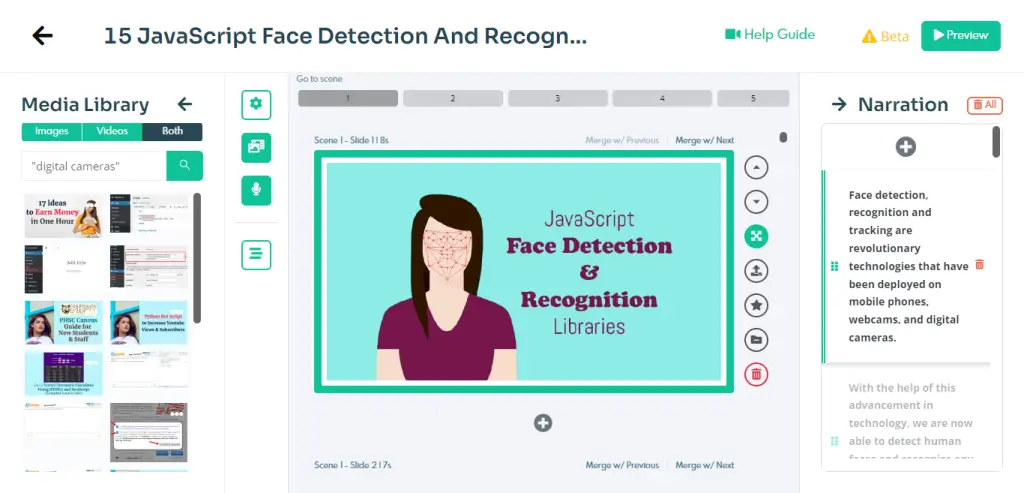
Anyways, In this tutorial, I’ll use Python 3 and OpenCV 3 to select all the images available in a specified folder and then combine them into an MP4 video.
The source code of image to video converter tool is written in a very simple and easy manner. So, that even beginner Python developers can understand and use it in their projects.
So, let’s have a look at the Python source code of images to video converter.
Image to Video Converter Tool Source Code
#!/usr/local/bin/python3 import cv2 import argparse import os # Construct the argument parser and parse the arguments ap = argparse.ArgumentParser() ap.add_argument("-ext", "--extension", required=False, default='png', help="extension name. default is 'png'.") ap.add_argument("-o", "--output", required=False, default='output.mp4', help="output video file") args = vars(ap.parse_args()) # Arguments dir_path = '.' ext = args['extension'] output = args['output'] images = [] for f in os.listdir(dir_path): if f.endswith(ext): images.append(f) # Determine the width and height from the first image image_path = os.path.join(dir_path, images[0]) frame = cv2.imread(image_path) cv2.imshow('video',frame) height, width, channels = frame.shape # Define the codec and create VideoWriter object fourcc = cv2.VideoWriter_fourcc(*'mp4v') # Be sure to use lower case out = cv2.VideoWriter(output, fourcc, 20.0, (width, height)) for image in images: image_path = os.path.join(dir_path, image) frame = cv2.imread(image_path) out.write(frame) # Write out frame to video cv2.imshow('video',frame) if (cv2.waitKey(1) & 0xFF) == ord('q'): # Hit `q` to exit break # Release everything if job is finished out.release() cv2.destroyAllWindows() print("The output video is {}".format(output))
Final Words
I think this code is very self-explanatory. Still, I’ve added a few comments to help you understand what’s going on.
If you have any questions regarding this images to video converter tool then let me know in the comments below.