In this tutorial, I will give you the complete JavaScript source code of the Google Maps Distance Matrix API example. You will learn how Google Maps Distance Matrix API measures the distance and calculates the journey duration between multiple locations (origins and destinations).
First of all, create a new HTML5 file on your computer and copy/paste the below source code into it.
JavaScript Google Maps Distance Matrix API Example
index.html
Important Note: On line # 6, replace ###YOUR_API_KEY###
text with your actual Google Maps API key. Otherwise, this code will not work.
<html> <head> <title>JavaScript Google Maps Distance Matrix API Example</title> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <script defer src="https://maps.googleapis.com/maps/api/js?libraries=places&language=en&key=###YOUR_API_KEY###" type="text/javascript"></script> <link href="https://netdna.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css" id="bootstrap-css" rel="stylesheet"/> </head> <body> <div class="container"> <div class="row"> <div class="jumbotron"> <h1>JavaScript Google Maps Distance Matrix API Example</h1> </div> <div class="col-md-6"> <form id="distance_form"> <div class="form-group"> <label>Origin: </label> <input class="form-control" id="from_places" placeholder="Enter a location" /> <input id="origin" name="origin" required="" type="hidden" /> </div> <div class="form-group"> <label>Destination: </label> <input class="form-control" id="to_places" placeholder="Enter a location" /> <input id="destination" name="destination" required="" type="hidden" /> </div> <input class="btn btn-primary" type="submit" value="Calculate" /> </form> <div id="result"> <ul class="list-group"> <li id="mile" class=" list-group-item d-flex justify-content-between align-items-center " > Distance In Mile : </li> <li id="kilo" class=" list-group-item d-flex justify-content-between align-items-center " > Distance is Kilo: </li> <li id="text" class=" list-group-item d-flex justify-content-between align-items-center " > IN TEXT: </li> <li id="minute" class=" list-group-item d-flex justify-content-between align-items-center " > IN MINUTES: </li> <li id="from" class=" list-group-item d-flex justify-content-between align-items-center " > FROM: </li> <li id="to" class=" list-group-item d-flex justify-content-between align-items-center " > TO: </li> </ul> </div> </div> </div> </div> <script> $(function () { // add input listeners google.maps.event.addDomListener(window, "load", function () { var from_places = new google.maps.places.Autocomplete( document.getElementById("from_places") ); var to_places = new google.maps.places.Autocomplete( document.getElementById("to_places") ); google.maps.event.addListener( from_places, "place_changed", function () { var from_place = from_places.getPlace(); var from_address = from_place.formatted_address; $("#origin").val(from_address); } ); google.maps.event.addListener( to_places, "place_changed", function () { var to_place = to_places.getPlace(); var to_address = to_place.formatted_address; $("#destination").val(to_address); } ); }); // calculate distance function calculateDistance() { var origin = $("#origin").val(); var destination = $("#destination").val(); var service = new google.maps.DistanceMatrixService(); service.getDistanceMatrix( { origins: [origin], destinations: [destination], travelMode: google.maps.TravelMode.DRIVING, unitSystem: google.maps.UnitSystem.IMPERIAL, // miles and feet. // unitSystem: google.maps.UnitSystem.metric, // kilometers and meters. avoidHighways: false, avoidTolls: false, }, callback ); } // get distance results function callback(response, status) { if (status != google.maps.DistanceMatrixStatus.OK) { $("#result").html(err); } else { var origin = response.originAddresses[0]; console.log(origin); var destination = response.destinationAddresses[0]; console.log(destination); if (response.rows[0].elements[0].status === "ZERO_RESULTS") { $("#result").html( "Better get on a plane. There are no roads between " + origin + " and " + destination ); } else { var distance = response.rows[0].elements[0].distance; console.log(distance); var duration = response.rows[0].elements[0].duration; console.log(duration); console.log(response.rows[0].elements[0].distance); var distance_in_kilo = distance.value / 1000; // the kilom var distance_in_mile = distance.value / 1609.34; // the mile console.log(distance_in_kilo); console.log(distance_in_mile); var duration_text = duration.text; var duration_value = duration.value; $("#mile").html( `Distance in Miles: ${distance_in_mile.toFixed(2)}` ); $("#kilo").html( `Distance in Kilometre: ${distance_in_kilo.toFixed(2)}` ); $("#text").html(`Distance in Text: ${duration_text}`); $("#minute").html(`Distance in Minutes: ${duration_value}`); $("#from").html(`Distance From: ${origin}`); $("#to").text(`Distance to: ${destination}`); } } } // print results on submit the form $("#distance_form").submit(function (e) { e.preventDefault(); calculateDistance(); }); }); </script> </body> </html>
Project Screenshot
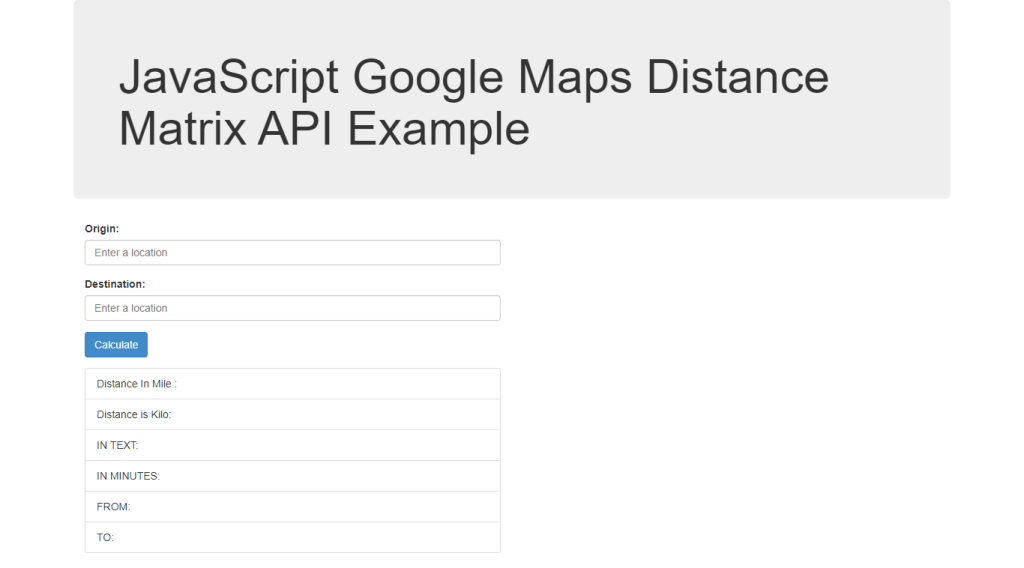