In this tutorial, I will guide you on how to add a Facebook login system to your website or app.
You might have seen many apps that accept login from different social media platforms (i.e. Facebook, Google, Microsoft, GitHub, Twitter, etc.). Basically, a social login enables us to quickly register new users in our app without forcing them to fill lengthy signup forms.
Today, we will use Facebook login JavaScript SDK and Graph API to implement Facebook login. The complete sample code of JavaScript Facebook Login with examples is given below.
Add Facebook Developer App
At first, you need to add a new app in the Facebook developers section. So, let’s add a new app and get its app ID.
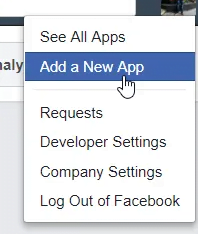
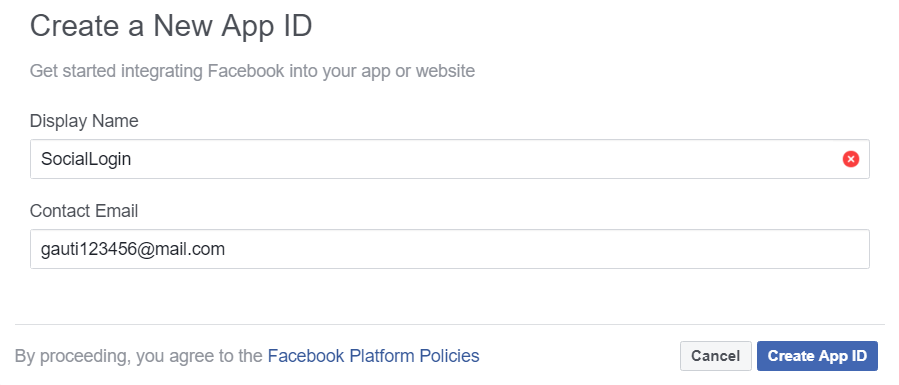
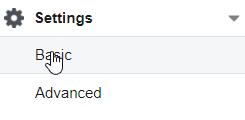
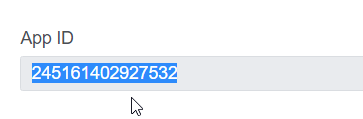
Now copy this app ID and store it somewhere. We will use this app ID in our application.
Facebook Login JavaScript Sample Code
After that, create a new file index.html for the login and logout button.
index.html
<!DOCTYPE html> <html> <head> <title>Facebook Login using JavaScript and jQuery</title> <meta charset="UTF-8"> </head> <style> </style> <script src="init.js"></script> <body> <button id="login">Login with Facebook</button> <button style="display:none;" id="logout">Logout</button> <div id="status"> </div> </body> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <script src="script.js"></script> </html>
init.js
Here you need to copy/paste your app ID which we generated earlier at the specified location (i.e. Code Line # 13).
(function(d, s, id) { var js, fjs = d.getElementsByTagName(s)[0]; if (d.getElementById(id)) return; js = d.createElement(s); js.id = id; js.src = "https://connect.facebook.net/en_US/sdk.js"; fjs.parentNode.insertBefore(js, fjs); }(document, 'script', 'facebook-jssdk')); // initialize the facebook sdk window.fbAsyncInit = function() { FB.init({ appId : '#### YOUR APP ID ########', cookie : true, // enable cookies to allow the server to access // the session xfbml : true, // parse social plugins on this page version : 'v3.1' // The Graph API version to use for the call }); }
script.js
$(document).ready(function(){ // add event listener on the login button $("#login").click(function(){ facebookLogin(); }); // add event listener on the logout button $("#logout").click(function(){ $("#logout").hide(); $("#login").show(); $("#status").empty(); facebookLogout(); }); function facebookLogin() { FB.getLoginStatus(function(response) { console.log(response); statusChangeCallback(response); }); } function statusChangeCallback(response) { console.log(response); if(response.status === "connected") { $("#login").hide(); $("#logout").show(); fetchUserProfile(); } else{ // Logging the user to Facebook by a Dialog Window facebookLoginByDialog(); } } function facebookLoginByDialog() { FB.login(function(response) { statusChangeCallback(response); }, {scope: 'public_profile,email'}); } function fetchUserProfile() { console.log('Welcome! Fetching your information.... '); FB.api('/me?fields=id,name,email,gender,birthday', function(response) { console.log(response); console.log('Successful login for: ' + response.name); var profile = `<h1>Welcome {response.name}<h1> <h2>Your email is ${response.email}</h2> <h3>Your Birthday is ${response.birthday}</h3>`; $("#status").append(profile); }); } function facebookLogout() { FB.logout(function(response) { statusChangeCallback(response); }); } }