What is Pokemon Encounter Calculator?
Pokemon Encounter Calculator is an online tool that takes Map Parameters like Version, Current map, Encounter Type, etc. as input and displays available Pokemon along with the likelihood of it appearing. It also shows an encounter rate which simply means how fast a Pokémon will appear (this only slows down if you use repels).
How to Make Pokemon Encounter Calculator?
In this tutorial, I will show you how to make your own Pokemon Encounter Calculator using C#. You don’t need to be a programmer to follow along with this tutorial. You can simply copy/paste or download the source code that I have provided in this guide.
You will get the complete source code of the Pokemon Encounter Calculator as well as a guide on how to use this software tool.
Supported Platforms
- Windows
- Mac
- Linux
- Online Web-based
Let’s get started making Pokemon Encounter Calc.
Pokemon Encounter Calculator Source Code
Program.cs
using System; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; using System.Windows.Forms; using System.Globalization; namespace PokemonEncCalc { static class Program { internal const string VERSION = "5.13"; internal const Version STARTING_VERSION = Version.Gold; /// <summary> /// The main entry point for the application. /// </summary> [STAThread] static void Main() { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); if (Properties.Settings.Default.Language == 0) detectSystemLanguage(); // Load data PokemonTables.PopulatePokemonTables(); Utils.loadEncounterSlotData(); Utils.initializeMoves(); Application.Run(new frmMainPage()); } static void detectSystemLanguage() { if (CultureInfo.CurrentUICulture.Name.StartsWith("fr")) Properties.Settings.Default.Language = 2; else if (CultureInfo.CurrentUICulture.Name.StartsWith("chs")) Properties.Settings.Default.Language = 8; else if (CultureInfo.CurrentUICulture.Name.StartsWith("cht")) Properties.Settings.Default.Language = 9; else Properties.Settings.Default.Language = 1; } } }
Settings.cs
namespace PokemonEncCalc.Properties { // This class allows you to handle specific events on the settings class: // The SettingChanging event is raised before a setting's value is changed. // The PropertyChanged event is raised after a setting's value is changed. // The SettingsLoaded event is raised after the setting values are loaded. // The SettingsSaving event is raised before the setting values are saved. internal sealed partial class Settings { public Settings() { // // To add event handlers for saving and changing settings, uncomment the lines below: // // this.SettingChanging += this.SettingChangingEventHandler; // // this.SettingsSaving += this.SettingsSavingEventHandler; // } private void SettingChangingEventHandler(object sender, System.Configuration.SettingChangingEventArgs e) { // Add code to handle the SettingChangingEvent event here. } private void SettingsSavingEventHandler(object sender, System.ComponentModel.CancelEventArgs e) { // Add code to handle the SettingsSaving event here. } } }
Fixed20_12.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace PokemonEncCalc { class Fixed20_12 { public int Number { get; set; } public int IntegerPart { get; set; } public int DecimalPart { get; set; } public bool Sign { get; set; } // True = positive, false = negative public Fixed20_12(int value = 0) { Sign = (value & 0x80000000) != 0; IntegerPart = value & 0x7FFFF000 >> 12; DecimalPart = value & 0x00000FFF; } public Fixed20_12(decimal value = 0) { Sign = value >= 0; value = Math.Abs(value); IntegerPart = (int)Math.Floor(value); Number = (int)(value * 4096); } public Fixed20_12(int integerPart, int decimalPart) { bool positive = integerPart >= 0; if ((integerPart & 0x7FFFFFFF) >= 0x00080000) integerPart = 0x0007FFFF; decimalPart &= 0x00000FFF; Number = (integerPart << 20) + decimalPart; unchecked { if (!positive) Number |= (int)0x80000000; } } public static Fixed20_12 operator+(Fixed20_12 left, Fixed20_12 right) { return new Fixed20_12(left.Number + right.Number); } } }
Screenshots
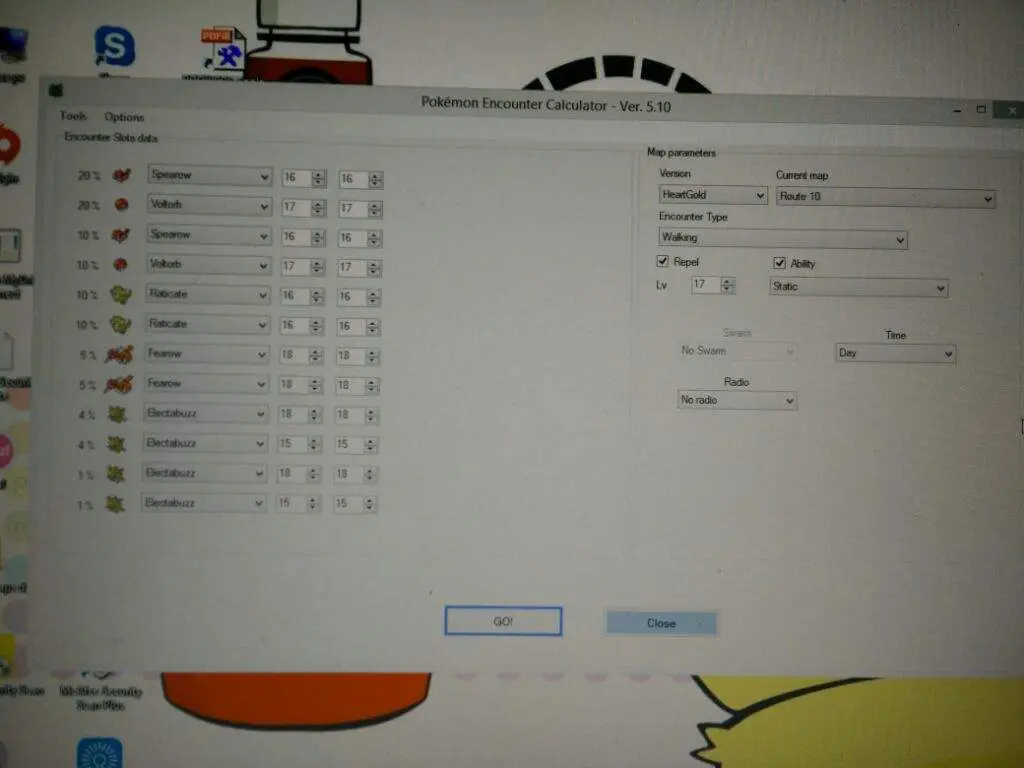
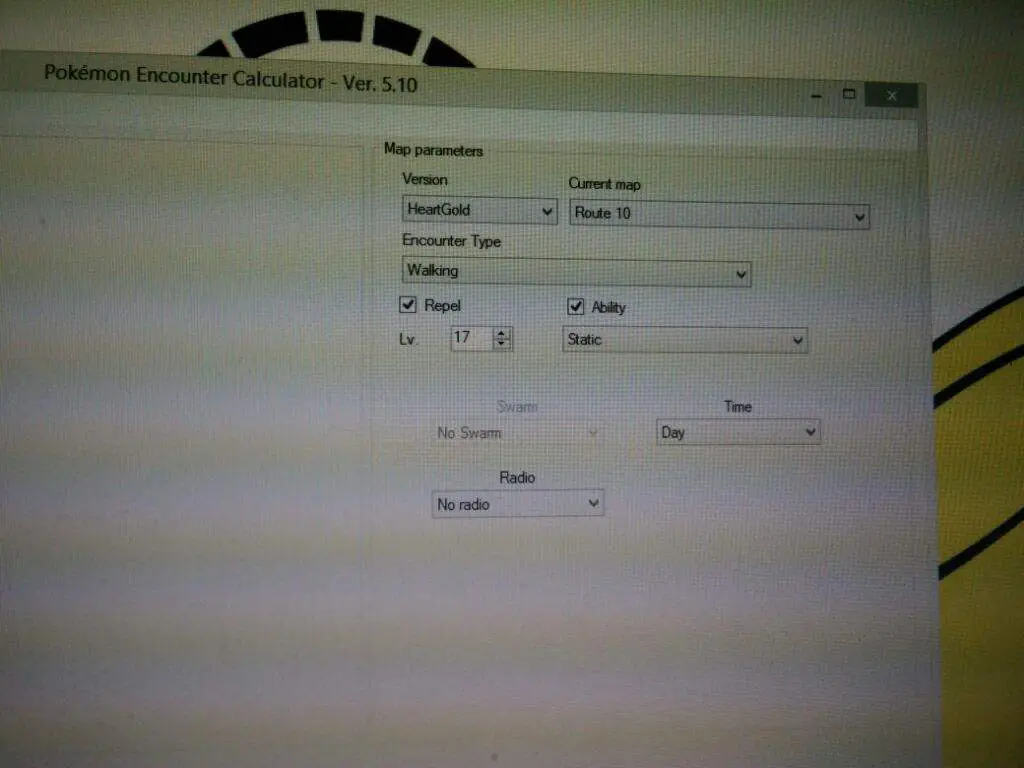
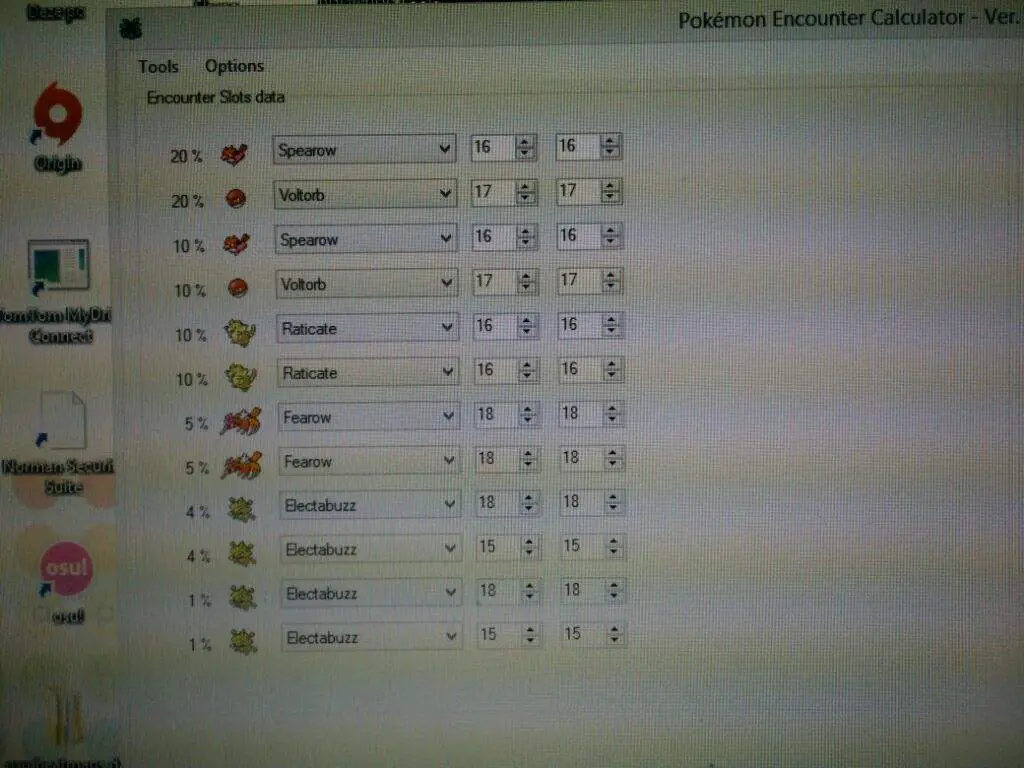
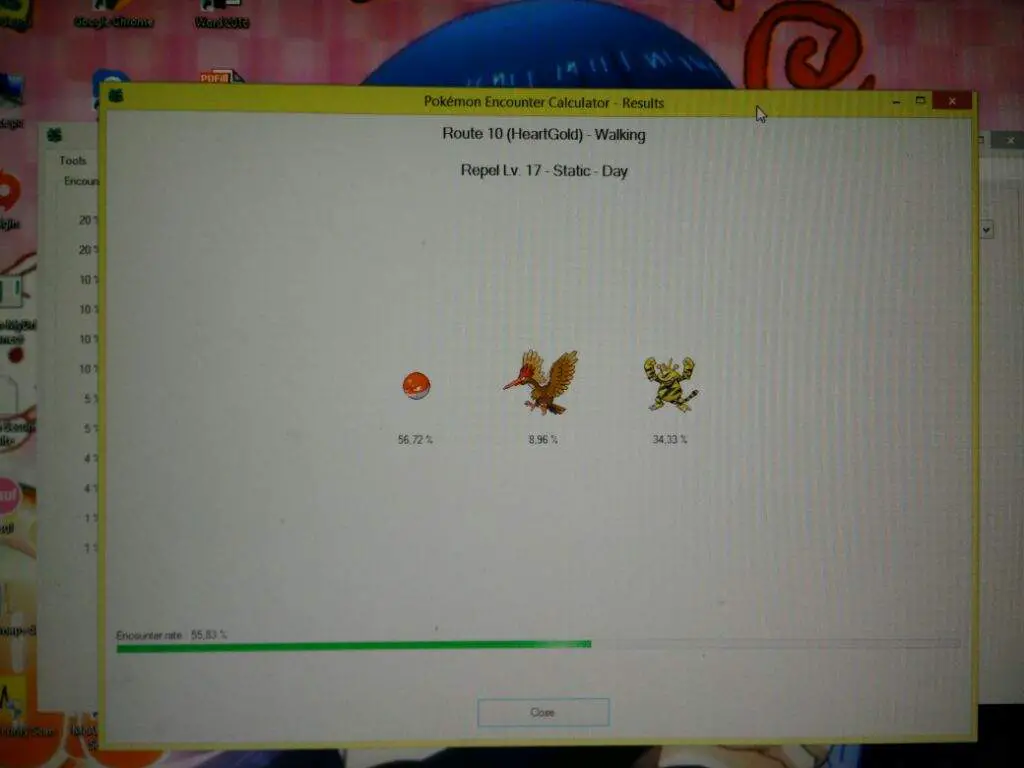
Download
Download the full source code of the Pokemon Encounter Calculator.