In this tutorial, you will learn how to download a PDF file from a URL using jQuery and AJAX. We will also use some HTML5 and core JavaScript code to build the application.
The complete source code of the PDF downloader developed using jQuery and AJAX is given below.
To get started, simply create a new HTML file index.html
and copy/paste the below-mentioned code into it.
index.html
<button type="button" id="download_pdf_file">Download PDF File!</button> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script> <script> $('#download_pdf_file').on('click', function () { $.ajax({ url: 'https://s3-us-west-2.amazonaws.com/s.cdpn.io/172905/test.pdf', method: 'GET', xhrFields: { responseType: 'blob' }, success: function (data) { var a = document.createElement('a'); var url = window.URL.createObjectURL(data); a.href = url; a.download = 'my_file.pdf'; document.body.append(a); a.click(); a.remove(); window.URL.revokeObjectURL(url); } }); }); </script>
Code Explanation
- At first, we will create a button using HTML. The user will click this button to download the file.
- Load the jQuery using CDN.
- Attach an onClick event to the “Download PDF File!” button. This method will be executed whenever the button is pressed.
- Now make an AJAX call to the desired PDF file using the built-in
ajax()
method of jQuery.
Run the Project
In order to see our code in action, go ahead and open the index.html
file in your favorite web browser.
The output will look something like this screenshot.

Here, you need to click the “Download PDF File!” button.
When you click this button, the PDF file will automatically start downloading on your PC.
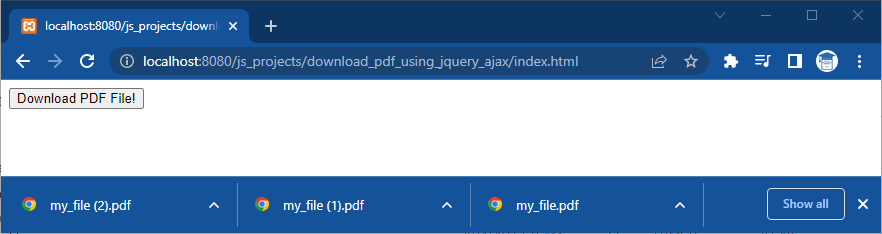